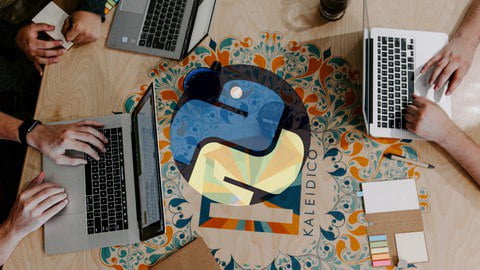
Table of Contents
Python coding questions and answers for Apptitude on topics various topics covering.
- Write a function that takes a list of integers and returns the sum of the integers.
Copy codedef sum_list(numbers):
total = 0
for number in numbers:
total += number
return total
- Write a function that takes a list of strings and returns a new list with all strings converted to uppercase.
Copy codedef to_upper(strings):
return [string.upper() for string in strings]
- Write a fAunction that takes a list of integers and returns a new list with only the odd integers.
Copy codedef odd_numbers(numbers):
return [number for number in numbers if number % 2 == 1]
- Write a function that takes a list of integers and returns the maximum value.
Copy codedef max_value(numbers):
return max(numbers)
- Write a function that takes a list of integers and returns True if the list is sorted in ascending order, False otherwise.
Copy codedef is_sorted(numbers):
return numbers == sorted(numbers)
- Write a function that takes a string and returns a new string with all vowels removed.
Copy codedef remove_vowels(string):
vowels = 'aeiouAEIOU'
return ''.join([char for char in string if char not in vowels])
- Write a function that takes a list of strings and a string and returns the index at which the string is found in the list, or -1 if the string is not found.
Copy codedef find_index(strings, target):
try:
return strings.index(target)
except ValueError:
return -1
- Write a function that takes a list of integers and returns the mean (average) of the list.
Copy codedef mean(numbers):
return sum(numbers) / len(numbers)
- Write a function that takes two lists of integers and returns a new list containing the elements that are present in both lists.
Copy codedef intersect(list1, list2):
return [value for value in list1 if value in list2]
- Write a function that takes a list of integers and returns a new list with the integers sorted in descending order.
Copy codedef sort_descending(numbers):
return sorted(numbers, reverse=True)
Python coding questions on swapping
- Write a function that takes two integers and swaps their values.
Copy codedef swap(a, b):
a, b = b, a
return a, b
- Write a function that takes a list of integers and swaps the first and last elements of the list.
Copy codedef swap_first_last(numbers):
if len(numbers) > 1:
numbers[0], numbers[-1] = numbers[-1], numbers[0]
return numbers
- Write a function that takes a dictionary and swaps the keys and values.
Copy codedef swap_keys_values(dictionary):
return {value: key for key, value in dictionary.items()}
- Write a function that takes a list of strings and swaps the first and last elements of each string.
Copy codedef swap_first_last_string(strings):
return [string[-1] + string[1:-1] + string[0] if len(string) > 1 else string for string in strings]
Python coding questions on String operations
- Write a function that takes a string and returns a new string with all vowels removed.
Copy codedef remove_vowels(string):
vowels = 'aeiouAEIOU'
return ''.join([char for char in string if char not in vowels])
- Write a function that takes a string and returns a new string with all occurrences of a specific character removed.
Copy codedef remove_char(string, char):
return ''.join([c for c in string if c != char])
- Write a function that takes a string and returns True if the string is a palindrome (reads the same backwards and forwards), False otherwise.
Copy codedef is_palindrome(string):
return string == string[::-1]
- Write a function that takes a string and returns a new string with all characters in the string converted to lowercase and all spaces removed.
Copy codedef lowercase_no_spaces(string):
return ''.join([c for c in string.lower() if c != ' '])
- Write a function that takes a string and returns a new string with the characters in the string reversed.
Copy codedef reverse_string(string):
return string[::-1]
Python coding questions on sorting
- Write a function that takes a list of integers and returns the list sorted in ascending order.
Copy codedef sort_ascending(numbers):
return sorted(numbers)
- Write a function that takes a list of strings and returns the list sorted in alphabetical order.
Copy codedef sort_alphabetically(strings):
return sorted(strings)
- Write a function that takes a list of dictionaries and returns the list sorted by the value of a specific key in the dictionaries.
Copy codedef sort_by_key(dictionaries, key):
return sorted(dictionaries, key=lambda x: x[key])
- Write a function that takes a list of integers and returns the list sorted in descending order.
Copy codedef sort_descending(numbers):
return sorted(numbers, reverse=True)
- Write a function that takes a list of tuples and returns the list sorted by the second element in each tuple.
Copy codedef sort_by_second_element(tuples):
return sorted(tuples, key=lambda x: x[1])
Python coding questions on time complexity
- Write a function that takes a list of integers and returns the sum of the squares of the integers. The function should have a time complexity of O(n).
Copy codedef sum_square(numbers):
total = 0
for number in numbers:
total += number ** 2
return total
- Write a function that takes two strings and returns True if the strings are anagrams (contain the same characters), False otherwise. The function should have a time complexity of O(n).
Copy codedef is_anagram(string1, string2):
if len(string1) != len(string2):
return False
return sorted(string1) == sorted(string2)
- Write a function that takes a list of integers and returns the second-largest element in the list. The function should have a time complexity of O(n).
Copy codedef second_largest(numbers):
largest = max(numbers)
numbers.remove(largest)
return max(numbers)
- Write a function that takes a list of strings and returns the longest string in the list. The function should have a time complexity of O(n).
Copy codedef longest_string(strings):
longest = ''
for string in strings:
if len(string) > len(longest):
longest = string
return longest
- Write a function that takes a list of integers and returns True if the list contains any duplicate elements, False otherwise. The function should have a time complexity of O(n).
Copy codedef has_duplicates(numbers):
return len(numbers) != len(set(numbers))
Join us for Regular Updates
Telegram | Join Now |
Join Now | |
Join Now | |
Join Now | |
Join Now | |
Join our Telegram | connectkreations |
Recent Jobs And Internships
- Mastering Data Structures and Algorithms for Engineering Aptitude: Series 1Data Structures and Algorithms (DSA) are crucial for anyone looking to excel in the field of software engineering. Understanding DSA… Read more: Mastering Data Structures and Algorithms for Engineering Aptitude: Series 1
- TCS iON free course with certificate -Apply NowAbout this Course: The job market today is highly competitive, and young professionals need to equip themselves with essential employability… Read more: TCS iON free course with certificate -Apply Now
- Free Courses offered by Google with Certificate, Boosts Resume 100X | Learn NowFree Courses offered by Google with Certificate, Boosts Resume 100X | Learn Now. The following is a list of free… Read more: Free Courses offered by Google with Certificate, Boosts Resume 100X | Learn Now
- Top Common Interview Questions and Answers for 2023, Gain confidenceTop Common Interview Questions and Answers for 2023, Learn to Gain confidence Gain Confidence with this tips 1. Tell me… Read more: Top Common Interview Questions and Answers for 2023, Gain confidence
- Python coding questions and answers for ApptitudeTable of Contents Python coding questions and answers for Apptitude on topics various topics covering. Copy codedef mean(numbers): return sum(numbers)… Read more: Python coding questions and answers for Apptitude
- Questions on various topics in Java, along with their answersHere are five multiple choice questions on various topics in Java, along with their answers: Join us for Regular Updates… Read more: Questions on various topics in Java, along with their answers
- Write An Incredible Resume: The Golden Rules!In this article, we will look at the The Golden Rules of Writing the Perfect Resume. Understanding how to write… Read more: Write An Incredible Resume: The Golden Rules!
- Top Technologies and Domains to Learn for Excellent Career OpportunitiesDiscussion Top Technologies and Domain to learn for Best career opportunity. Read the details and choose the right career path.There… Read more: Top Technologies and Domains to Learn for Excellent Career Opportunities
- For Placement Preparation. Placement Cell
- FOR MORE COURSES. FREE COURSES
- FOR Job Updates. JOB AND INTERNSHIP UPADTES
- For Latest Trends in Technology. Technology Blog
- For Quotes and Poems. Quotes-Poems
About Connect Kreations
We the team Connect Kreations have started a Blog page which is eminently beneficial to all the students those who are seeking jobs and are eager to develop themselves in a related area. As the world is quick on uptake, our website also focuses on latest trends in recent technologies. We are continuously putting our efforts to provide you with accurate, best quality, and genuine information. Here we also have complete set of details on how to prepare aptitude, interview and more of such placement/ off campus placement preparation.
Connect Kreations is excited to announce the expansion of our services into the realm of content creation! We are now offering a wide range of creative writing services, including poetry, articles, and stories.
Whether you need a heartfelt poem for a special occasion, a thought-provoking article for your blog or website, or an engaging story to captivate your audience, our team of talented writers is here to help. We have a passion for language and a commitment to creating high-quality content that is both original and engaging.
Our services are perfect for individuals, businesses, and organizations looking to add a touch of creativity and personality to their content. We are confident that our unique perspectives and diverse backgrounds will bring a fresh and exciting voice to your project.
Thank you for choosing Connect Kreations for your content creation needs. We look forward to working with you and helping you to bring your vision to life!
The website is open to all and we want all of you to make the best use of this opportunity and get benefit from it..🤓
- Our Websites: Connect Kreations